주요 흐름
1. 사용자가 로그인한 경우, 세션에서 사용자 정보를 가져옴.
2. API 호출을 위한 JSON 데이터 구성하여 connect 함수를 통해 서버에 전송.
3. 응답 데이터를 JSON으로 파싱하여 처리.
4. 성공적인 경우, 인증키를 받아서 자동으로 로그인을 처리하는 페이지를 생성.
5. 잘못된 사용자나 오류가 발생한 경우 리다이렉트 처리.
코드
<%--import 영역--%>
<%!
// 세션에서 SessionBean 객체를 얻어오는 메서드
public static SessionBean session() {
return (SessionBean)Beans.get(SessionBean.class);
}
/****
* 서버에 JSON 데이터를 POST 요청으로 전송하고 응답을 받아오는 메서드
* - url: 요청할 서버의 URL
* - json: 전송할 JSON 데이터
* - mode: "https" 사용 여부
* - sendCharSet: 전송할 데이터의 문자셋
* - receiveCharSet: 응답 받을 데이터의 문자셋
* - propertyMap: HTTP 헤더에 추가할 속성들
* - readTime: 응답을 기다리는 최대 시간(타임아웃)
****/
public static String connect(String url, String json, String mode, String sendCharSet, String receiveCharSet, Map<String, String> propertyMap, String readTime) throws Exception {
PrintWriter postReq = null;
BufferedReader postRes = null;
String resultJson = null;
StringBuilder resultBuffer = new StringBuilder();
try {
// URL 객체 생성
URL connectUrl = new URL(url);
// HTTPS 모드인지 확인하여 HTTPS 또는 HTTP 연결 생성
if ("https".equals(mode)) {
HttpsURLConnection con = (HttpsURLConnection) connectUrl.openConnection();
con.setRequestMethod("POST"); // POST 메서드 설정
con.setDoOutput(true); // 서버에 데이터를 전송할 수 있도록 설정
con.setUseCaches(false); // 캐시 사용 안 함
con.setDoInput(true); // 서버로부터 입력 받음
con.setConnectTimeout(5000); // 연결 타임아웃 5초 설정
if (readTime != null) {
con.setReadTimeout(Integer.parseInt(readTime)); // 응답 대기 시간 설정
}
// propertyMap에 있는 헤더들을 요청에 추가
if (propertyMap != null) {
for (String key : propertyMap.keySet()) {
con.setRequestProperty(key, propertyMap.get(key));
}
}
// JSON 데이터를 서버로 전송
postReq = new PrintWriter(new OutputStreamWriter(con.getOutputStream(), sendCharSet));
postReq.write(json); // JSON 데이터 쓰기
postReq.flush(); // 버퍼에 있는 데이터를 전송
// 서버로부터 응답을 수신
postRes = new BufferedReader(new InputStreamReader(con.getInputStream(), receiveCharSet));
while ((resultJson = postRes.readLine()) != null) {
resultBuffer.append(resultJson); // 응답 데이터를 resultBuffer에 추가
}
con.disconnect(); // 연결 종료
} else {
HttpURLConnection con = (HttpURLConnection) connectUrl.openConnection();
con.setRequestMethod("POST");
con.setDoOutput(true);
con.setUseCaches(false);
con.setConnectTimeout(5000);
if (readTime != null) {
con.setReadTimeout(Integer.parseInt(readTime));
}
if (propertyMap != null) {
for (String key : propertyMap.keySet()) {
con.setRequestProperty(key, propertyMap.get(key));
}
}
postReq = new PrintWriter(new OutputStreamWriter(con.getOutputStream(), sendCharSet));
postReq.write(json);
postReq.flush();
postRes = new BufferedReader(new InputStreamReader(con.getInputStream(), receiveCharSet));
while ((resultJson = postRes.readLine()) != null) {
resultBuffer.append(resultJson);
}
con.disconnect();
}
} catch (SocketTimeoutException e) {
throw e; // 타임아웃 예외 처리
} catch (Exception e) {
throw e; // 기타 예외 처리
} finally {
// 자원 해제
if (postReq != null) postReq.close();
if (postRes != null) postRes.close();
}
return resultBuffer.toString(); // 서버 응답 반환
}
%>
<%
try {
// 세션에 사용자 정보가 있는지 확인
if (session().getUserInfo() != null) {
String userNumb = session().getUserInfo().getUserId();
// 사용자 정보가 없을 경우 리다이렉트
if (userNumb == null || "".equals(userNumb)) {
response.sendRedirect("https://sendRedirectPage.co.kr");
return;
}
// JSON 요청 데이터 구성
JSONObject tranreq = new JSONObject();
tranreq.put("SVC_CD", "apiServiceCode"); // API 서비스 코드
tranreq.put("BIZ_NO", "111"); // 사업자번호
tranreq.put("USER_ID", userNumb); // 사용자 아이디
String platFormProtocol = "https"; // HTTPS 사용 여부
String url = "https://url.co.kr"; // 요청할 서버의 기본 URL
// API 요청 후 응답 수신
String strResultData = connect(url + "/apiservice.jsp", "JSONData=" + URLEncoder.encode(tranreq.toJSONString(), "EUC-KR"), platFormProtocol, "EUC-KR", "EUC-KR", null, "5000");
// 응답 데이터 디코딩
strResultData = URLDecoder.decode(strResultData, "EUC-KR");
// 응답 데이터를 JSON 파싱
JSONParser parser = new JSONParser();
JSONObject jsonResponse = (JSONObject) parser.parse(strResultData);
String resultCode = (String) jsonResponse.get("resultCode"); // 결과 코드
String resultMessage = (String) jsonResponse.get("resultMSG"); // 결과 메시지
String Key = (String) jsonResponse.get("KEY"); // 키
// 응답이 정상적인 경우 처리
if(("0000".equals(resultCode)) && ("정상".equals(resultMessage))) {
%>
<html>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script language="javascript" type="text/javascript">
function doLogin() {
frm.action = "https://actionUrl.co.kr/loginApi.act";
frm.target = "_self";
frm.method = "post";
frm.submit(); // 로그인 API로 폼 전송
}
// 페이지가 로드되면 자동으로 doLogin 호출
$(document).ready(function() {
doLogin();
});
</script>
<body>
<form id="frm" name="frm" >
<input type="hidden" id="BIZ_NO" name="BIZ_NO" value="111"/><br/>
<input type="hidden" id="USER_ID" name="USER_ID" value="<%=userNumb%>"/><br/>
<input type="hidden" id="KEY" name="KEY" value="<%=Key%>"/><br/>
<input type="hidden" id="PAGE_GB" name="SVCPAGE_GB_CD" value=""/><br/>
</form>
</body>
</html>
<%
}
} else {
//요청 클라이언트가 로그인 되어 있지 않을 경우 로그인 페이지로 Redirect
String nextUrl = "https://login.co.kr";
String scheme = request.getScheme();
String str = request.getServerName();
response.sendRedirect(nextUrl);
}
} catch (Exception e) {
out.print("Error: " + e.getMessage());
}
%>
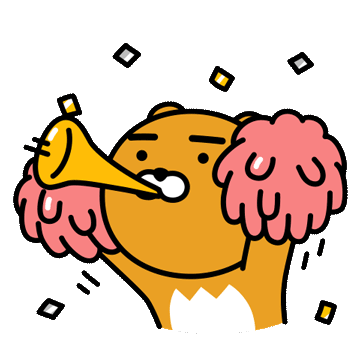
반응형
'Dev > Spring&Java' 카테고리의 다른 글
[Java] Base64 인코딩/디코딩: JDK 1.7과 JDK 1.8에서의 차이점 (0) | 2024.10.04 |
---|---|
[Java] 지역 변수와 스코프(Scope) (0) | 2024.08.14 |
The type className is already defined 에러 해결 방법 (0) | 2024.06.25 |
The selected JRE does not support the current compiler compliance level of 1.8 (0) | 2024.04.09 |
[Java] 이클립스 JDK 버전 설정/변경하기 (0) | 2024.04.09 |